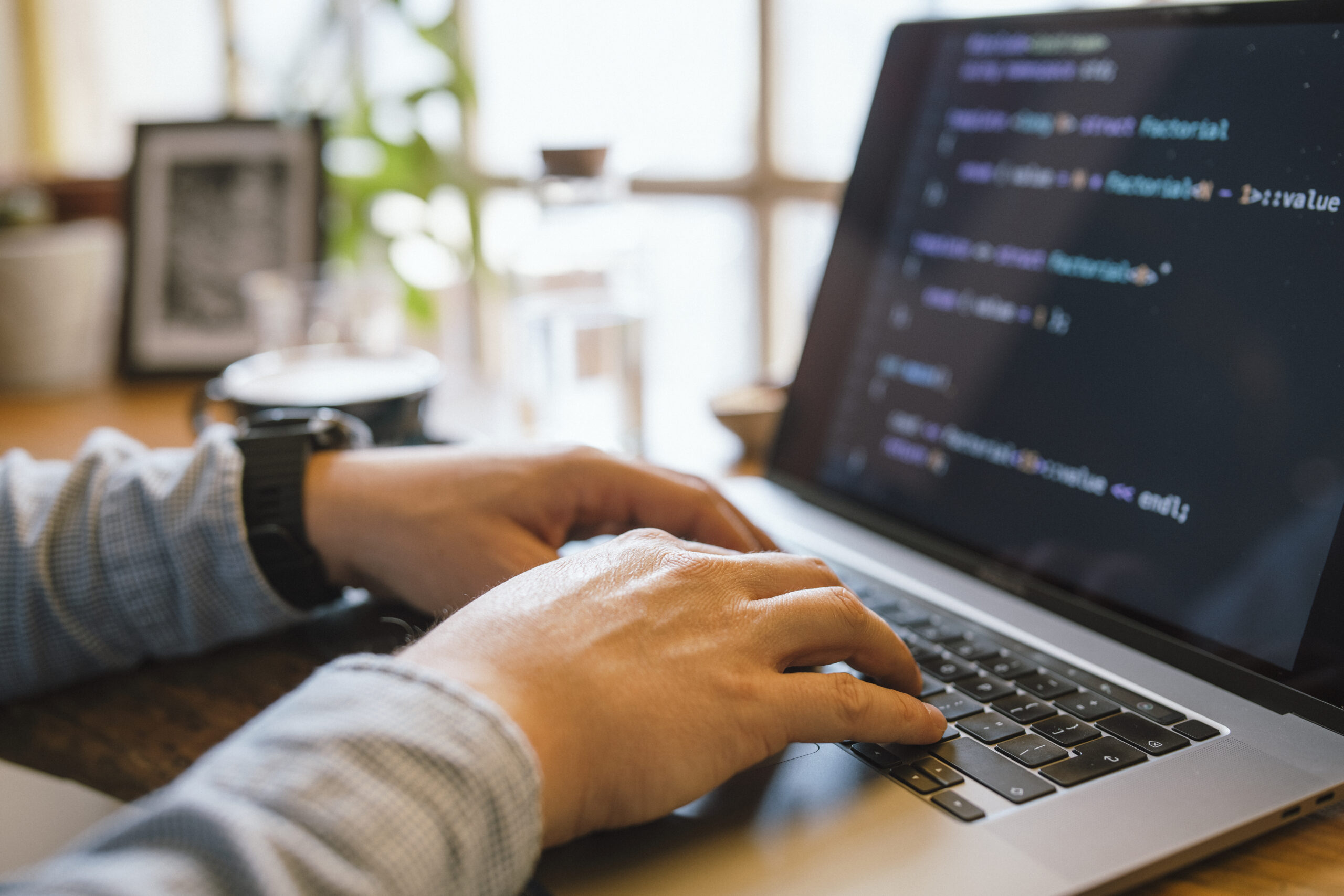
Debugging is one of the most vital — nonetheless often ignored — capabilities inside a developer’s toolkit. It is not almost repairing damaged code; it’s about knowledge how and why matters go Improper, and Finding out to Imagine methodically to unravel complications competently. Whether or not you are a novice or possibly a seasoned developer, sharpening your debugging capabilities can help save hrs of stress and substantially improve your efficiency. Here i will discuss quite a few procedures that will help builders amount up their debugging video game by me, Gustavo Woltmann.
Grasp Your Equipment
One of many quickest ways developers can elevate their debugging abilities is by mastering the tools they use everyday. Though producing code is one particular Element of progress, being aware of the best way to interact with it correctly through execution is Similarly critical. Modern day development environments occur Outfitted with potent debugging abilities — but lots of developers only scratch the surface of what these instruments can do.
Choose, one example is, an Built-in Progress Environment (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These equipment enable you to set breakpoints, inspect the value of variables at runtime, step by way of code line by line, as well as modify code over the fly. When employed correctly, they Enable you to observe accurately how your code behaves in the course of execution, which happens to be priceless for monitoring down elusive bugs.
Browser developer resources, like Chrome DevTools, are indispensable for entrance-end developers. They assist you to inspect the DOM, check community requests, view real-time functionality metrics, and debug JavaScript inside the browser. Mastering the console, resources, and network tabs can switch frustrating UI issues into manageable jobs.
For backend or system-degree builders, applications like GDB (GNU Debugger), Valgrind, or LLDB supply deep control in excess of running processes and memory management. Mastering these tools could possibly have a steeper learning curve but pays off when debugging efficiency difficulties, memory leaks, or segmentation faults.
Further than your IDE or debugger, turn out to be relaxed with Variation Regulate systems like Git to comprehend code heritage, obtain the precise moment bugs were launched, and isolate problematic improvements.
Finally, mastering your tools indicates going past default configurations and shortcuts — it’s about acquiring an personal expertise in your development environment to ensure that when concerns occur, you’re not missing at the hours of darkness. The greater you are aware of your applications, the greater time you may devote solving the actual problem rather than fumbling through the process.
Reproduce the condition
The most essential — and sometimes ignored — actions in effective debugging is reproducing the problem. Right before leaping into your code or building guesses, developers require to create a dependable natural environment or state of affairs wherever the bug reliably appears. Without reproducibility, correcting a bug gets a recreation of chance, usually leading to squandered time and fragile code adjustments.
The first step in reproducing a challenge is collecting as much context as possible. Talk to issues like: What actions led to The problem? Which atmosphere was it in — enhancement, staging, or output? Are there any logs, screenshots, or error messages? The greater depth you have, the much easier it turns into to isolate the precise disorders beneath which the bug takes place.
As soon as you’ve gathered ample information, try and recreate the trouble in your neighborhood surroundings. This may suggest inputting a similar info, simulating identical user interactions, or mimicking method states. If The problem seems intermittently, think about producing automated exams that replicate the sting circumstances or point out transitions involved. These exams not simply assist expose the challenge but will also protect against regressions in the future.
At times, The problem may very well be atmosphere-distinct — it'd take place only on certain working programs, browsers, or less than specific configurations. Making use of instruments like Digital machines, containerization (e.g., Docker), or cross-browser screening platforms is often instrumental in replicating these types of bugs.
Reproducing the issue isn’t only a phase — it’s a way of thinking. It necessitates persistence, observation, plus a methodical tactic. But as you can consistently recreate the bug, you're currently halfway to fixing it. Using a reproducible circumstance, You may use your debugging applications more effectively, test prospective fixes properly, and converse additional Plainly with the staff or people. It turns an summary grievance right into a concrete problem — and that’s in which developers prosper.
Browse and Have an understanding of the Mistake Messages
Mistake messages will often be the most beneficial clues a developer has when a little something goes Erroneous. In lieu of observing them as aggravating interruptions, builders must discover to treat mistake messages as direct communications in the system. They often tell you exactly what took place, the place it occurred, and sometimes even why it took place — if you understand how to interpret them.
Begin by studying the information thoroughly and in comprehensive. Quite a few developers, specially when beneath time strain, glance at the 1st line and right away start building assumptions. But deeper during the error stack or logs could lie the legitimate root lead to. Don’t just copy and paste error messages into search engines like google — browse and realize them initial.
Crack the mistake down into parts. Could it be a syntax mistake, a runtime exception, or even a logic mistake? Does it place to a specific file and line quantity? What module or purpose triggered it? These inquiries can guide your investigation and position you towards the accountable code.
It’s also handy to be aware of the terminology of the programming language or framework you’re employing. Mistake messages in languages like Python, JavaScript, or Java frequently observe predictable patterns, and Understanding to acknowledge these can dramatically hasten your debugging process.
Some problems are imprecise or generic, As well as in These situations, it’s crucial to examine the context in which the error transpired. Test related log entries, input values, and recent alterations during the codebase.
Don’t forget about compiler or linter warnings both. These normally precede larger concerns and provide hints about likely bugs.
Finally, mistake messages are not your enemies—they’re your guides. Mastering to interpret them the right way turns chaos into clarity, helping you pinpoint concerns more quickly, lessen debugging time, and turn into a additional economical and self-assured developer.
Use Logging Properly
Logging is The most highly effective instruments inside of a developer’s debugging toolkit. When made use of effectively, it provides real-time insights into how an application behaves, aiding you recognize what’s occurring beneath the hood with no need to pause execution or phase throughout the code line by line.
A superb logging approach starts off with recognizing what to log and at what amount. Prevalent logging degrees include things like DEBUG, Details, WARN, ERROR, and Lethal. Use DEBUG for comprehensive diagnostic information during development, Facts for normal functions (like productive commence-ups), WARN for possible issues that don’t crack the appliance, ERROR for precise challenges, and Deadly once the system can’t carry on.
Avoid flooding your logs with too much or irrelevant facts. Excessive logging can obscure crucial messages and slow down your procedure. Target crucial functions, state variations, enter/output values, and critical conclusion factors inside your code.
Structure your log messages Obviously and regularly. Involve context, for example timestamps, request IDs, and performance names, so it’s easier to trace difficulties in distributed units or multi-threaded environments. Structured logging (e.g., JSON logs) might make it even much easier to parse and filter logs programmatically.
All through debugging, logs Allow you to keep track of how variables evolve, what situations are achieved, and what branches of logic are executed—all without having halting This system. They’re Specifically valuable in generation environments the place stepping as a result of code isn’t achievable.
On top of that, use logging frameworks and resources (like Log4j, Winston, or Python’s logging module) that aid log rotation, filtering, and integration with monitoring dashboards.
Eventually, intelligent logging is about stability and clarity. That has a properly-assumed-out logging method, you may reduce the time it will take to identify challenges, acquire deeper visibility into your apps, and improve the Total maintainability and trustworthiness within your code.
Believe Just like a Detective
Debugging is not simply a technological task—it's a method of investigation. To correctly identify and resolve bugs, developers ought to solution the process like a detective fixing a thriller. This state of mind aids break down intricate difficulties into workable pieces and follow clues logically to uncover the root trigger.
Commence by accumulating proof. Think about the indications of the problem: error messages, incorrect output, or overall performance problems. Much like a detective surveys a crime scene, gather just as much appropriate data as it is possible to devoid of leaping to conclusions. Use logs, examination situations, and consumer reviews to piece with each other a clear image of what’s taking place.
Subsequent, form hypotheses. Ask yourself: What can be producing this behavior? Have any modifications not too long ago been designed to your codebase? Has this situation transpired ahead of below comparable circumstances? The goal should be to slender down alternatives and detect opportunity culprits.
Then, take a look at your theories systematically. Try and recreate the challenge within a controlled atmosphere. If you suspect a selected operate or part, isolate it and verify if The difficulty persists. Just like a detective conducting interviews, inquire your code questions and Permit the outcome lead you nearer to the truth.
Pay near interest to compact information. Bugs often cover within the the very least anticipated places—just like a lacking semicolon, an off-by-a single mistake, or possibly a race condition. Be extensive and patient, resisting the urge to patch The problem without entirely understanding it. Momentary fixes may possibly disguise the true challenge, only for it to resurface later on.
Last of all, maintain notes on That which you tried and uncovered. Equally as detectives log their investigations, documenting your debugging procedure can preserve time for upcoming concerns and enable Other people recognize your reasoning.
By wondering like a detective, developers can sharpen their analytical techniques, approach issues methodically, and become more effective at uncovering hidden difficulties in elaborate methods.
Publish Assessments
Writing exams is one of the best solutions to boost your debugging capabilities and Over-all enhancement efficiency. Tests not just aid catch bugs early and also function a security net that gives you self-confidence when creating adjustments to the codebase. A properly-examined software is simpler to debug as it lets you pinpoint particularly where and when a problem occurs.
Start with device checks, which deal with unique capabilities or modules. These smaller, isolated assessments can speedily reveal irrespective of whether a selected bit of logic is Doing work as anticipated. Whenever a check fails, you instantly know where to appear, significantly lessening some time expended debugging. Device exams are Particularly helpful for catching regression bugs—issues that reappear just after Earlier getting set.
Next, combine integration exams and end-to-finish checks into your workflow. These enable be certain that numerous aspects of your software function together efficiently. They’re specifically useful for catching bugs that come about in sophisticated techniques with multiple parts or providers interacting. If something breaks, your assessments can tell you which Component of the pipeline failed and underneath what situations.
Crafting exams also forces you to definitely Imagine critically about your code. To check a characteristic thoroughly, you may need to understand its inputs, predicted outputs, and edge cases. This volume of knowing The natural way prospects to raised code framework and much less bugs.
When debugging an issue, producing a failing test that reproduces the bug could be a robust first step. After the take a look at fails regularly, it is possible to focus on fixing the bug and enjoy your examination go when The difficulty is resolved. This technique makes certain that the same bug doesn’t return Later on.
Briefly, crafting tests turns debugging from a annoying guessing activity into a structured and predictable approach—encouraging you capture much more bugs, more quickly plus much more reliably.
Choose Breaks
When debugging a tricky problem, it’s straightforward to become immersed in the challenge—observing your monitor for several hours, making an attempt Resolution immediately after Alternative. But one of the most underrated debugging tools is simply stepping away. Taking breaks aids you reset your brain, lessen stress, and sometimes see The problem from a new point of view.
When you are far too near to the code for way too prolonged, cognitive tiredness sets in. You could possibly start off overlooking clear problems or misreading code that you just wrote just hrs earlier. Within this condition, your brain gets to be less efficient at trouble-resolving. A brief walk, a coffee crack, or maybe switching to a distinct activity for 10–quarter-hour can refresh your concentration. Many builders report obtaining the basis of a more info problem when they've taken time and energy to disconnect, letting their subconscious work during the qualifications.
Breaks also support stop burnout, especially all through more time debugging classes. Sitting before a display screen, mentally stuck, is not simply unproductive but in addition draining. Stepping away means that you can return with renewed Vitality as well as a clearer mindset. You would possibly abruptly notice a lacking semicolon, a logic flaw, or perhaps a misplaced variable that eluded you prior to.
When you’re stuck, a very good guideline should be to set a timer—debug actively for forty five–60 minutes, then have a 5–10 moment break. Use that point to move all over, stretch, or do a little something unrelated to code. It might experience counterintuitive, Specially under restricted deadlines, but it really truly causes more quickly and more practical debugging In the end.
Briefly, taking breaks just isn't an indication of weakness—it’s a sensible strategy. It provides your Mind space to breathe, enhances your standpoint, and assists you stay away from the tunnel eyesight that often blocks your progress. Debugging can be a psychological puzzle, and rest is part of fixing it.
Study From Each and every Bug
Each individual bug you encounter is much more than simply A short lived setback—it's an opportunity to expand for a developer. Whether it’s a syntax error, a logic flaw, or even a deep architectural challenge, every one can instruct you some thing useful in case you make an effort to reflect and examine what went Mistaken.
Get started by asking your self several essential issues as soon as the bug is fixed: What prompted it? Why did it go unnoticed? Could it have been caught earlier with better methods like unit testing, code testimonials, or logging? The solutions typically reveal blind spots within your workflow or knowing and allow you to Create more powerful coding behavior relocating forward.
Documenting bugs can also be a great habit. Keep a developer journal or manage a log in which you Observe down bugs you’ve encountered, the way you solved them, and That which you uncovered. After a while, you’ll start to see patterns—recurring issues or popular faults—which you could proactively keep away from.
In group environments, sharing what you've acquired from the bug along with your peers is usually In particular strong. No matter if it’s by way of a Slack information, a brief compose-up, or A fast know-how-sharing session, aiding Other people steer clear of the very same problem boosts workforce effectiveness and cultivates a stronger Mastering tradition.
Extra importantly, viewing bugs as lessons shifts your mindset from annoyance to curiosity. As opposed to dreading bugs, you’ll commence appreciating them as critical areas of your development journey. In spite of everything, a number of the most effective builders are not those who write best code, but those who continually learn from their problems.
Eventually, Each and every bug you take care of adds a different layer to your talent set. So following time you squash a bug, have a moment to mirror—you’ll occur away a smarter, a lot more able developer because of it.
Conclusion
Increasing your debugging skills normally takes time, observe, and patience — even so the payoff is large. It makes you a more productive, self-assured, and able developer. The subsequent time you might be knee-deep in a mysterious bug, bear in mind: debugging isn’t a chore — it’s a chance to be improved at what you do.